Webサービスを運営するのであれば、少なくともユーザーアカウントと管理者アカウントの最低2つは要する必要があるかと思います。
そこで、今回はDeviseを使ってユーザーアカウント(user)と管理者アカウント(admin)を分けて作成、認証(マルチログイン)する方法についてまとめてみました。
参考になれば幸いです。
開発環境
- Ruby 3.1.2
- Ruby on Rails 7.0.4
- tailwindcss 3.1.8
- M1 Macbook Air 2020
- mac OS Monterey (ver. 12.4)
- ターミナル bash (Rosetta 2 使用)
deviseで複数アカウントを作成する手順
それでは順番に解説していきます。
Deviseのインストール
まずはGemfile
に下記のように記述します。
gem 'devise'
以下のコマンドでgemをインストールします。
$ bundle install
続いて、以下のコマンドでDevise本体をインストールします。
$ rails g devise:install
Deviseのインストールが完了すると、ターミナルに以下のような表示が出ます。
===============================================================================
Depending on your application's configuration some manual setup may be required:
1. Ensure you have defined default url options in your environments files. Here
is an example of default_url_options appropriate for a development environment
in config/environments/development.rb:
config.action_mailer.default_url_options = { host: 'localhost', port: 3000 }
In production, :host should be set to the actual host of your application.
* Required for all applications. *
2. Ensure you have defined root_url to *something* in your config/routes.rb.
For example:
root to: "home#index"
* Not required for API-only Applications *
3. Ensure you have flash messages in app/views/layouts/application.html.erb.
For example:
<p class="notice"><%= notice %></p>
<p class="alert"><%= alert %></p>
* Not required for API-only Applications *
4. You can copy Devise views (for customization) to your app by running:
rails g devise:views
* Not required *
===============================================================================
Devise設定ファイルの変更
config/initializers/devise.rb
の中の、「# config.scoped_views = false」の部分を以下のように書き換えます。
config.scoped_views = true
Deviseはデフォルトで全てのモデルに同じビューを使用しているため、上記のようにすることでモデルごとに別々のビューを使用できるようになります。(adminとuserそれぞれ別のビューを使用できるようになる)
「admin」と「user」モデルの作成
以下のコマンドを実行して「admin」モデルと「user」モデルを作成します。
$ rails g devise admin
$ rails g devise user
モデル作成時にマイグレーションファイルが作成されるので、変更がなければマイグレーションを実行します。
カラムの追加やConfirmableの設定などをしておきたい場合は、マイグレーション実行前に以下のようにマイグレーションファイルを書き換えておきましょう。
# frozen_string_literal: true
class DeviseCreateUsers < ActiveRecord::Migration[7.0]
def change
create_table :users do |t|
## Database authenticatable
t.string :name # ←nameカラムを追加する場合は追記する
t.string :email, null: false, default: ""
t.string :encrypted_password, null: false, default: ""
## Recoverable
t.string :reset_password_token
t.datetime :reset_password_sent_at
## Rememberable
t.datetime :remember_created_at
## Trackable
# t.integer :sign_in_count, default: 0, null: false
# t.datetime :current_sign_in_at
# t.datetime :last_sign_in_at
# t.string :current_sign_in_ip
# t.string :last_sign_in_ip
# Confirmable # ↓メール認証を有効にする場合はコメントアウトを外す
t.string :confirmation_token
t.datetime :confirmed_at
t.datetime :confirmation_sent_at
t.string :unconfirmed_email # Only if using reconfirmable
## Lockable
# t.integer :failed_attempts, default: 0, null: false # Only if lock strategy is :failed_attempts
# t.string :unlock_token # Only if unlock strategy is :email or :both
# t.datetime :locked_at
t.timestamps null: false
end
add_index :users, :email, unique: true
add_index :users, :reset_password_token, unique: true
# add_index :users, :confirmation_token, unique: true
# add_index :users, :unlock_token, unique: true
end
end
書き換えたらマイグレーションを実行します。
$ rails db:migrate
コントローラーの作成
続いて、以下のコマンドでコントローラーを作成します。
$ rails g devise:controllers admins
$ rails g devise:controllers users
(※コントローラーなので複数形で!)
ビューの作成
以下のコマンドでビューを作成します(こちらも複数形で)。
$ rails g devise:views admins
$ rails g devise:views users
ルーティング設定
最後にルーティングの設定を行います。
現状のルーティング設定は以下のようになっているかと思います。
Rails.application.routes.draw do
・・・
devise_for :users
devise_for :admins
end
しかし、この状態のままだと個別に追加したコントローラーを参照してくれません(Deviseデフォルトのコントローラーを参照してしまう)。
そこで、以下のように記述を変更することで各モデルに対応したコントローラーを参照するようになります。
Rails.application.routes.draw do
・・・
devise_for :admins, controllers: {
# ↓ローカルに追加されたコントローラーを参照する(コントローラー名: "コントローラーの参照先")
registrations: "admins/registrations",
sessions: "admins/sessions",
passwords: "admins/passwords",
confirmations: "admins/confirmations"
}
devise_for :users, controllers: {
# ↓ローカルに追加されたコントローラーを参照する(コントローラー名: "コントローラーの参照先")
registrations: "users/registrations",
sessions: "users/sessions",
passwords: "users/passwords",
confirmations: "users/confirmations"
}
end
以上です。
Railsバージョン7でDeviseを利用する場合
現状(2023年1月11日時点)では最新バージョンのRails7にDeviseがまだ対応していないため、このままではいくつかエラーが発生してしまいます。
Rails7で開発を進めている方は、下記記事の「Undefined method ‘user_url’」エラーへの対処とControllerをカスタマイズの項目を参考に、設定の追加とコントローラーのカスタマイズを行っておいてください。
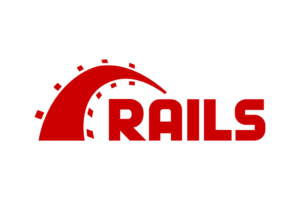
参考資料
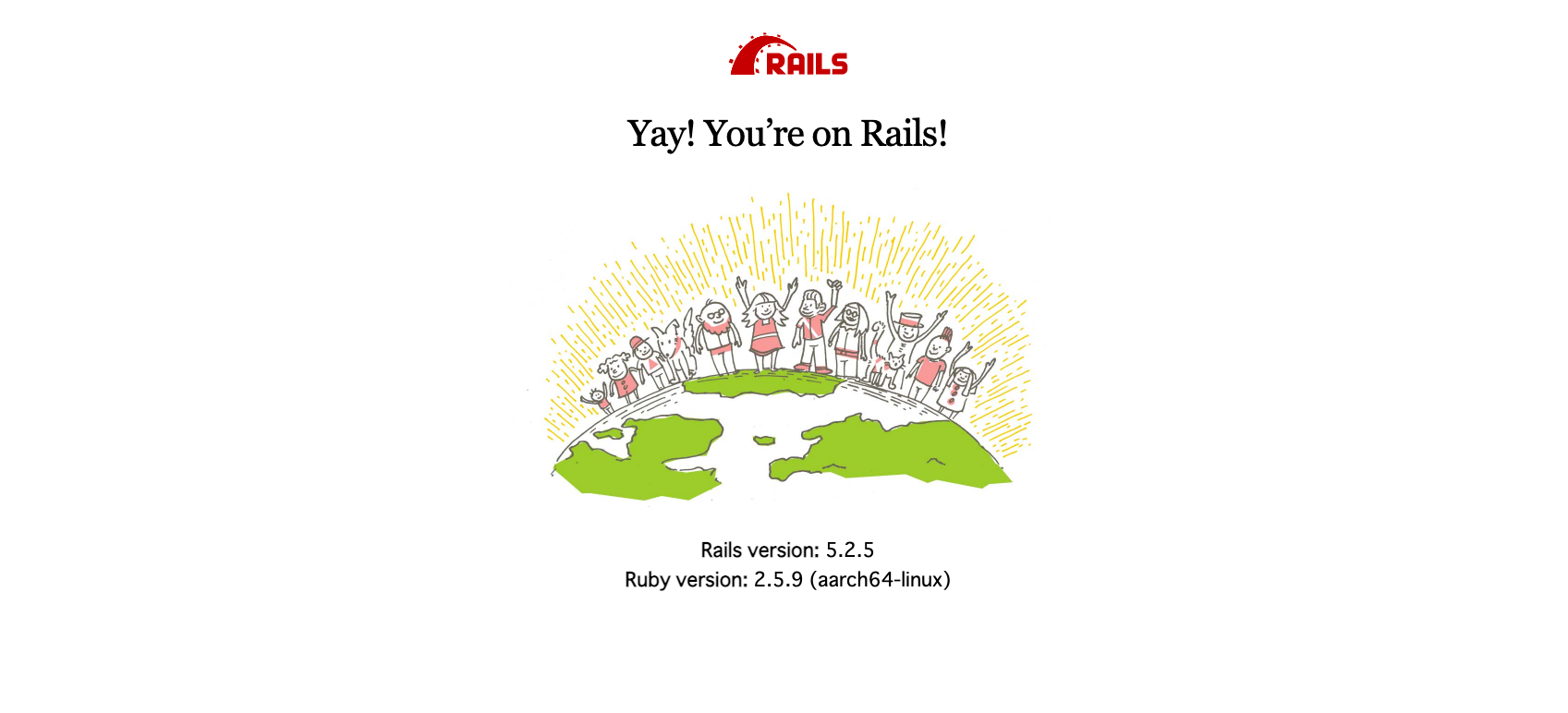
コメント