ユーザー認証としてDeviseを用いたRailsアプリケーションに、ユーザー用のマイページを作ろうと思っていたのですが、
「そういえば、ユーザー名やプロフィールなどの作成、編集ってどうやるんだろう?」
と疑問に思ったので、調べてみたところ、Deviseを少々カスタマイズする必要がありました。
そこで、今回はDeviseでユーザーのプロフィールを作成、表示および編集ができるようカスタマイズする方法についてまとめました。
どなたかの参考になれば幸いです。
開発環境
- Ruby 3.1.2
- Ruby on Rails 7.0.4.3
- Bootstrap 5.2.3
- Devise 4.9.2
- M1 Macbook Air 2020
- mac OS Monterey (ver. 12.4)
Devise でユーザーのプロフィール作成、表示および編集ができるようカスタマイズ
Deviseを導入していることを前提として話を進めていくので、事前にインストールしておいてください。
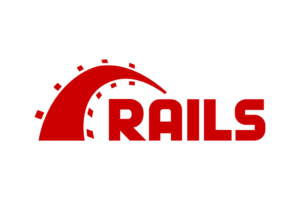
Userモデルの作成
以下のコマンドでUserモデルを作成します。
$ rails g devise user
Viewの作成
$ rails g devise:views users
上記コマンドを実行することで、app/views/users
以下にDevise用のviewが自動生成されます。
Controllerの作成
$ rails g devise:controllers users
上記コマンドを実行することで、app/controllers/users
以下にDevise用のコントローラーが自動生成されます。
後ほど、コントローラーの一部をカスタマイズします。
userテーブルにカラムを追加する
Deviseにはデフォルトでnameカラムとprofileカラムが用意されていないので、マイグレーションファイルを作成してカラムを追加します。
$ rails g migration add_profile_to_users
マイグレーションファイルが生成されたら、以下のように記述↓
class AddAvatarProfileToUsers < ActiveRecord::Migration[7.0]
def change
add_column :users, :name, :string # ユーザー名
add_column :users, :profile, :text # プロフィール
end
end
マイグレーションを実行してカラムを作成します。
$ rails db:migrate
userテーブルにnameとprofileカラムが追加されていることを確認しましょう。
deviseコントローラーにストロングパラメータを追加
先ほど追加したカラムのストロングパラメータを、application_controller.rb
に追加します。
class ApplicationController < ActionController::Base
# deviseコントローラーにストロングパラメータを追加する
before_action :configure_permitted_parameters, if: :devise_controller?
protected
def configure_permitted_parameters
# ユーザー登録時にnameのストロングパラメータを追加(サインアップ時にnameを入力する場合は追記)
devise_parameter_sanitizer.permit(:sign_up, keys: [:name])
# ユーザー編集時にnameとprofileのストロングパラメータを追加
devise_parameter_sanitizer.permit(:account_update, keys: [:name, :profile])
end
end
僕の場合、ユーザー登録時はメールアドレスとパスワードの入力のみで、登録後のユーザー編集時にnameとprofileの内容を追加、変更できるようにしたいので、:account_update
のパラメータのみ追加することにしました。
devise_parameter_sanitizer.permit(:account_update, keys: [:name, :profile])
ユーザー登録画面とユーザー編集画面の作成
ユーザー登録画面と編集画面を作成します。
まずは、ユーザー登録画面から。
<h2>Sign up</h2>
<%= form_for(resource, as: resource_name, url: registration_path(resource_name)) do |f| %>
<%= render "devise/shared/error_messages", resource: resource %>
<!-- 名前の入力フォームを追加(登録時にユーザー名を入力させたい場合) -->
<div class="field">
<%= f.label :name %><br />
<%= f.text_field :name, autofocus: true %>
</div>
<div class="field">
<%= f.label :email %><br />
<%= f.email_field :email, autofocus: true, autocomplete: "email" %>
</div>
<div class="field">
<%= f.label :password %>
<% if @minimum_password_length %>
<em>(<%= @minimum_password_length %> characters minimum)</em>
<% end %><br />
<%= f.password_field :password, autocomplete: "new-password" %>
</div>
<div class="field">
<%= f.label :password_confirmation %><br />
<%= f.password_field :password_confirmation, autocomplete: "new-password" %>
</div>
<div class="actions">
<%= f.submit "Sign up" %>
</div>
<% end %>
<%= render "devise/shared/links" %>
続いては、ユーザー編集画面(プロフィール編集画面)を作成します。
編集画面では、nameとprofileの入力フォームを追加し、デフォルトで記述してあったパスワード確認用のフォームを削除しています。
<h2>Edit <%= resource_name.to_s.humanize %></h2>
<%= form_for(resource, as: resource_name, url: registration_path(resource_name), html: { method: :put }) do |f| %>
<%= devise_error_messages! %>
<!-- 名前の入力フォームを追加 -->
<div class="field">
<%= f.label :name %><br />
<%= f.text_field :name, autofocus: true %>
</div>
<div class="field">
<%= f.label :email %><br />
<!-- emailを編集できないようにしたい場合は「disabled: true」オプションを追記する -->
<%= f.email_field :email, autofocus: true, autocomplete: "email" %>
</div>
<!-- ユーザー編集時にプロフィールを入力できるようにフォームを追加 -->
<div class="field">
<%= f.label :profile %><br />
<%= f.text_area :profile, autofocus: true %>
</div>
<div class="actions">
<%= f.submit "Update" %>
</div>
<% end %>
<%= link_to "Back", :back %>
プロフィール画面の作成
続いては、プロフィール画面(マイページ)の作成をしていきます。
以下のコマンドを実行し、マイページ用のview(show.html.erb)を作成します。
$ rails g controller Users show
続いて、users_controller.rb
に以下を追記します↓
class UsersController < ApplicationController
def show
@user = current_user
end
end
先ほど作成したviewに、プロフィールの内容を記述して完成です。
<h1>プロフィール</h1>
<h3>ユーザー名</h3>
<%= @user.name %>
<h3>メールアドレス</h3>
<%= @user.email %>
<h3>プロフィール</h3>
<%= @user.profile %>
ルーティング設定
先ほどローカルに追加したコントローラーを参照するよう、ルーティング設定を行います。
devise_for :users, controllers: { registrations: 'users/registrations' }
get "users/profile" => "users#show"
これで、app/controllers/users/registrations_controller.rb
の変更内容を反映してくれるようになります。
また、users/profile
にアクセスすると、current_userのプロフィール画面が表示されるようになります。
パスワードなしでユーザー情報を編集できるようにする
Deviseの仕様で、ユーザー情報の変更にはパスワードの入力が必要になるので、ユーザービリティ向上のためパスワードなしでユーザー情報を編集できるようにしていきます。
registrations_controller.rb
に以下を追記します。
class RegistrationsController < Devise::RegistrationsController
protected
def update_resource(resource, params)
resource.update_without_password(params)
end
end
これで、パスワードなしでプロフィールの編集ができるようになるはずです。
これで一通り完成ですが、一つだけ問題点があるので次の章で解説します。
プロフィール編集後にマイページへリダイレクトする方法
Deviseの仕様上、デフォルトのままだとユーザー情報(プロフィール)をアップデートしたとき、自動的にトップページにリダイレクトされてしまいます。
そのままでも良いかもしれませんが、普通はプロフィールを更新したら一旦プロフィール画面を確認したいと思うものですよね。
そこで、プロフィールを更新後はプロフィール画面(マイページ)にリダイレクトされるよう変更します。
リダイレクト先を変更するためには、registrations_controller.rb
に以下の内容を追記すればOKです。
class RegistrationsController < Devise::RegistrationsController
protected
def update_resource(resource, params)
resource.update_without_password(params)
end
############## ↓追記 ################
def after_update_path_for(resource)
# 自分で設定した「マイページ」へのパス
users_profile_path
end
####################################
end
上記のusers_profile_path
はルーティング設定で設定したマイページへのパス(users/profile)なので、ご自身のルーティング設定内容を確認してから記述してください。
これで、プロフィールの更新後はマイページにリダイレクトされるはずです。
以上です。
参考資料
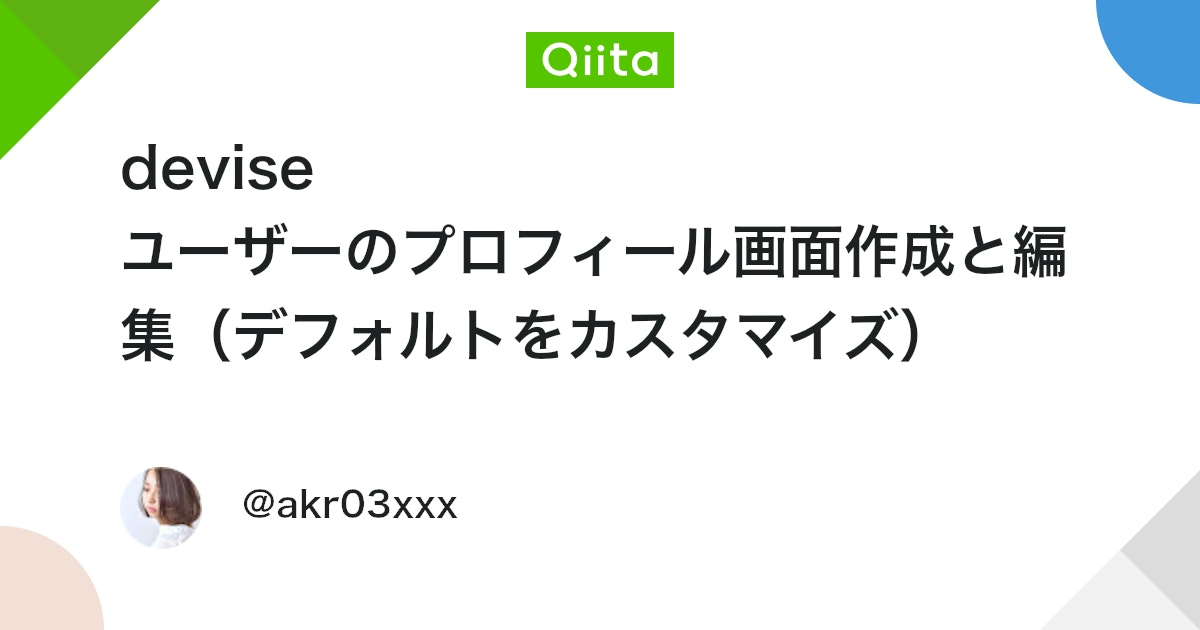
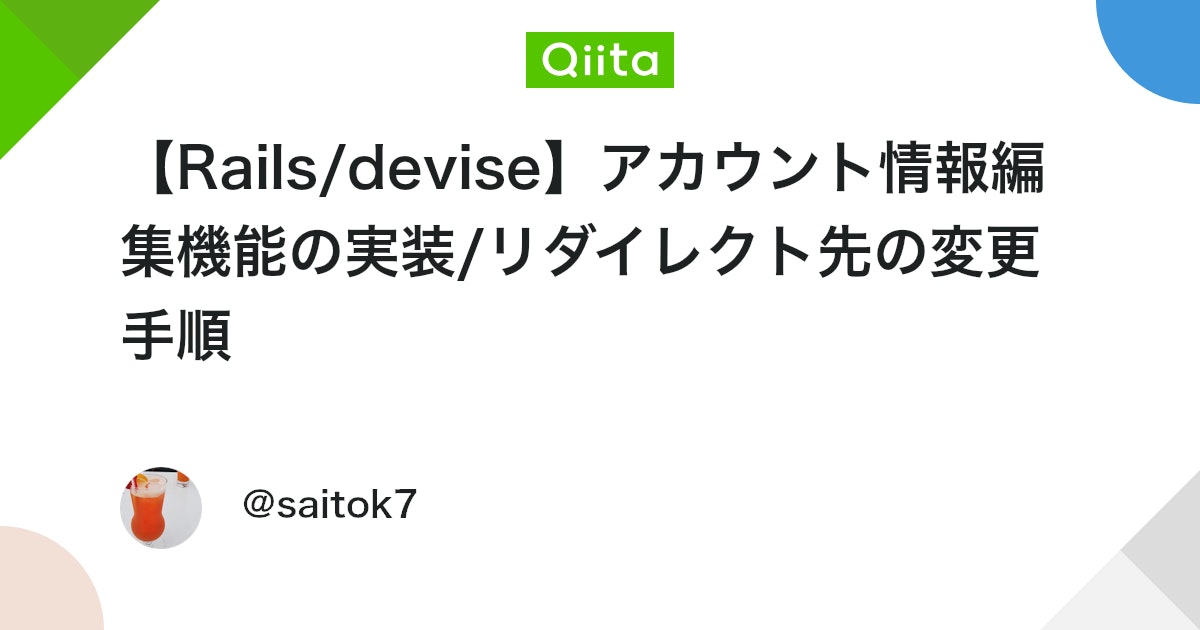
コメント